<script>
$(function() {
$("#article-content img").click(function() {
const t = $(this).next().next().next().offset();
if(t) {
$("body, html").animate({scrollTop: t}, 100);
} else {
return false;
}
});
});
</script>
アロー演算子=>はfunction()を省略することができ、上記ではfunction(t)をt => に変えています。その後、中括弧{}がありませんが、関数内で一つしか処理しない場合はif文やfor文みたく省くことができます。引数tはJSのthisを表すので、変数初期化を省いて、そこからjQueryを使わずにJSだけで攻めていきます。jQueryのnextはJSだとnextElementSiblingと長ったらしくなってしまうので、次の要素ではなく現在の画像の高さと下の要素の高さ分をスクロールするという形にしています。
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<script>
$(function() {
$("article-content img").click(t => {
$("body,html").animate({scrollTop: a.target.offsetTop + a.target.height + 68}, 100)
});
});
</script>
↓jQueryを除き、JavaScriptのみに構成することで一語一語長いですがライブラリを読み込まなくて済むのでとても早くなります。当然その場合jQueryを一切使わないよう、再構築しなければなりません。
<script>
const imgs = Array.from(document.getElementById("article-content").getElementsByTagName("img"));
imgs.forEach(i=>
i.addEventListener("click", t =>
t.target.offsetTop + t.target.height + 68));
</script>
↓画像タグにidを振って<img id="img01">、aタグで囲んでhrefを次の画像img02にすればJavaScriptさえいらなくなります。これが人類最速のスクロールJavaScriptだ!
そんなわけにはいかないので、jQueryの脱退に着手しました。
const a = document .getElementById`article`
, b = a && document .getElementById`article-content`.nextSibling.getAttribute`id`
, c = a && Array.from(a.getElementsByTagName`img`)
, d = a && Array.from(a.getElementsByClassName`rate`)
, e = a && Array.from(a.getElementsByClassName`i`)
, f = new XMLHttpRequest()
, g = document .getElementById`comments`
, h = g && Array.from(g.getElementsByClassName`cvs`)
, i = document .getElementById`gettags`
, j = document .getElementById`commentoptionshow`
, m = document .getElementsByClassName`beatbuttonshow`;
a && c.forEach (z=>z.addEventListener("click", x=>{
let y = x.target.offsetTop + x.target.height + 67;
scrollTo(0, y)
}
));
a && d.forEach (z=>z.addEventListener("click", x=>{
const v = x.target.classList.contains`bad` ? "bad" : "good";
f.onreadystatechange = function () {
f.readyState === 4 && f.status == 200 && (x.target.style.backgroundColor = "#2196f3",
x.target.style.color = "white",
x.target.children[1].textContent = "" + x.target.children[1].textContent - -1,
x.target.parentNode.children[0].setAttribute("disabled", true),
x.target.parentNode.children[1].setAttribute("disabled", true));
}
;
f.open("POST", "https://erozine.jp/vote", true);
f.setRequestHeader("Content-type", "application/x-www-form-urlencoded");
f.send(`box=${x.target.parentNode.id}&vote=${x.target.classList.contains("bad") ? "bad" : "good"}`);
}
));
a && e.forEach (z=>z.addEventListener("click", x=>{
x.target.style.pointerEvents = "none",
x.target.style.fontSize = "20px";
f.onreadystatechange = ()=>{
f.readyState === 4 && f.status == 200 && ((x.target.style.fontSize = "10px"),
x.target.classList.add("id"),
x.target.textContent = (x.target.textContent - -1))
}
;
f.open("POST", "https://erozine.jp/imgvote", true);
f.setRequestHeader("Content-type", "application/x-www-form-urlencoded");
f.send(`src=${x.target.parentNode.previousSibling.getAttribute("src")}&box=${b}`);
}
));
g && h.forEach (z=>z.addEventListener("click", x=>{
x.target.parentNode.children[0].style.pointerEvents = "none",
x.target.parentNode.children[1].style.pointerEvents = "none",
x.target.style.fontSize = "18px";
f.onreadystatechange = ()=>{
f.readyState === 4 && f.status == 200 && ((x.target.style.fontSize = "10px"),
x.target.classList.add("cid"),
x.target.textContent = (x.target.textContent - -1))
}
;
f.open("POST", "https://erozine.jp/commentvote", true);
f.setRequestHeader("Content-type", "application/x-www-form-urlencoded");
f.send(`cid=${x.target.parentNode.parentNode.getAttribute("id")}&v=${x.target.classList.contains("cg") ? "g" : "b"}`);
}
));
i.addEventListener("click", x=>{
f.onreadystatechange = function () {
if (f.readyState === 4 && f.status === 200) {
const u = document .createElement("ul")
, o = JSON.parse(f.responseText);
u.setAttribute("id", "tag-list");
u.classList.add("flex");
u.innerHTML = "";
Object.keys(o).forEach (k=>u.innerHTML += `<li><a href="/tag/${k}" rel="tag">${k}<br><span>${o[k]}</span></a></li>`);
document .getElementById("topnavi").parentNode.insertBefore(u, document .getElementById("topnavi").nextSibling);
}
}
;
f.open("GET", "https://erozine.jp/gettags.json", true);
f.setRequestHeader("Content-type", "application/x-www-form-urlencoded");
f.send(null);
}
);
j && j.addEventListener("click", x=>{
f.onreadystatechange = function () {
if (f.readyState === 4 && f.status === 200) {
x.target.remove();
document .getElementById`commentoption`.innerHTML = f.responseText;
}
}
;
f.open("GET", "https://erozine.jp/commentoption.html", true);
f.setRequestHeader("Content-type", "application/x-www-form-urlencoded");
f.send(null);
}
);
g && document .getElementById`commentbutton`.addEventListener("click", x=>{
const birthday = document .getElementsByName("birthday")[0].value
, na = document .getElementById`name`.value || ""
, body = document .getElementById`commentbody`.value;
let seieki;
if (seieki = document .getElementById`seieki`) {
seieki = seieki.value || null;
}
if (body) {
const cf = document .getElementById`commentform`;
f.onreadystatechange = ()=>{
if (f.readyState === 4 && f.status == 200) {
cf.remove();
document .getElementById`comments`.nextSibling.innerHTML = `<article class="comment"><div class="commentmeta"><span class="commentcurrent">YOU</span>${name} 1秒前 ID: 0x000000</div><div class="commentbody">${body}</div></article><div class="caution">コメントは投稿されました。リアルタイムで確認するには<a href="/beat">コメント掲示板</a>をご覧くださいませ。</div>`;
}
}
;
f.open("POST", "https://erozine.jp/pushcomment", true);
f.setRequestHeader("Content-type", "application/x-www-form-urlencoded");
f.send(`birthday=${birthday}&name=${name}&body=${body}&seieki=${seieki}`);
}
}
);
m && Array.from(m).forEach (z=>z.addEventListener("click", x=>{
const cf = document .getElementById`commentform`;
x.target.parentNode.appendChild(cf);
document .getElementById`lion`.value = cf.parentNode.getAttribute`id`;
cf.parentNode.parentNode.setAttribute("id", "comments");
document .getElementById`commentbody`.focus();
x.target.remove();
}
));
最後までお読みいただき有難うございました
Thank you for watching until the end
似たような記事





新着
相互リンク

新着
相互リンク

新着
相互リンク

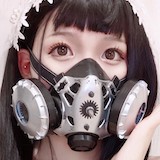

From teens and coeds to moms and mature ladies, from first-timers to experienced sluts, from virgins to pornstars, from solo models to couples, swingers and more – we offer you the whole variety of sex tube content adult industry produces and we put it out comfortably sorted and ready for playback for your maximum convenience. Just open a category you find intriguing or go to one of our top lists and click a video you like. <br> <br><a href=https://banginteens.com> Come get your daily dose of free sex tube videos with eager amateurs, hot face-sitting babes, big-breasted moms, agile teens and cum-hungry sluts</a> <br> <br>Come get your daily dose of free sex tube videos with eager amateurs, hot face-sitting babes, big-breasted moms, agile teens and cum-hungry sluts - https://banginteens.com(23件) 

